‘Word clouds’ is nothing but a way to use Python for visually displaying the frequency of words in a text dataset. There is a library called WorldCloud that we can use in Python to provide a quick overview of the most common words within the text, making it easy to identify key themes and trends. In this tutorial. we find out the command for installing the WordCloud library using PIP, a Python package manager and then we also demonstrate how to generate word clouds from text data.
Step 1: Get Python and PIP
It doesn’t matter which operating system you are using Windows, Linux, or MacOS; to follow this tutorial you must have Python already installed on your system along with PIP.
However, those who don’t have them already can see our article – Python & PIP install on Linux and Windows.
(optional) Also, it’s a good approach, if you use virtual environments to isolate your Python projects. You can create a virtual environment using tools like virtualenv or venv to install WordCloud within that environment.
So, those who want to set up a virtual environment can follow these steps otherwise move to the next one:
Install the Virtualenv package:
sudo apt-get install python3-venv
Use the given command and create a new Python virtual environment:
python3 -m venv testenv
Once the virtual environment is created, to activate it run:
source testenv/bin/activate
Note: testenv is our environment name you can give something else if you want:
Step 2: Installing the WordCloud using PIP
Before learning how to use WordClouds, let’s first install it. And the best way to install the Python library including WordCloud is PIP. So, open your system’s command terminal or prompt and run the given command.
pip install wordcloud --user
Wait for the installation process to complete. Once finished, you’ll be ready to create word clouds.

Step 3: Example to generate Word Cloud in Python
Here’s a simple Python program that demonstrates how to generate a word cloud using the WordCloud library:
Now, create a file to add the Python code where we import Wordcloud and other required libraries for creating a cloud of Words.
nano word.py
Add the below-given code in the file:
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# Sample text data
text_data = "This is a sample text data for generating a word cloud. Word clouds are fun and informative visualizations."
# Create a WordCloud object
wordcloud = WordCloud(width=800, height=800, background_color='white', min_font_size=10).generate(text_data)
# Display the word cloud using matplotlib
plt.figure(figsize=(8, 8), facecolor=None)
plt.imshow(wordcloud)
plt.axis("off")
plt.tight_layout(pad=0)
plt.show()
After adding the code, save the file by pressing Ctrl+X, type Y and hit the Enter key.
Now, run the Python file you have created using the given command:
python word.py
Note: If you get an error: raise ValueError(“Only supported for TrueType fonts”) ValueError: Only supported for TrueType fonts… Then don’t forget to upgrade your PIP and Pillow versions.
pip install --upgrade pip
pip install --upgrade Pillow
Here is the WordCloud output you will have on your screen.
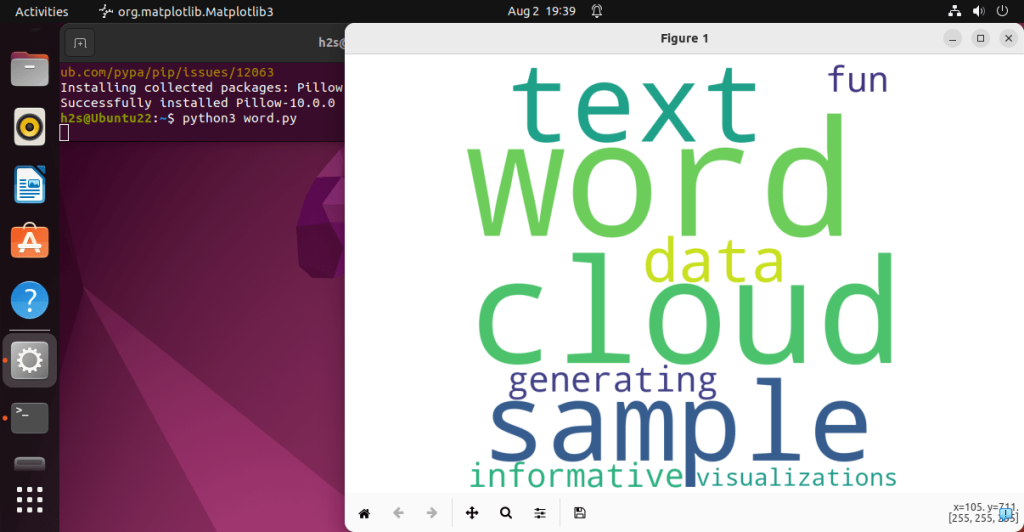
Explanation:
In this example, we first imported two necessary Python libraries – WordCloud and Matplotlib. After that, we have defined what text we want to use for creating a cloud of words. Following that, in the code, we create a WordCloud object, setting various parameters such as width, height, background color, and minimum font size.
The .generate() method of the WordCloud object is used to generate the word cloud from the provided text data. Finally, we use matplotlib to display the generated word cloud as an image without axes.
Remember, we just have given a simple example. WordCloud is not limited to that only. You can customize various aspects of the word cloud, such as colors, fonts, and masks as per your needs. Even, you can also read text data from a file or a more complex dataset to create more informative word clouds.
Here are a few customization options you can explore:
colormap
: Set the color map for the word cloud.font_path
: Specify the path to a custom font file.mask
: Use an image as a shape mask for the word cloud.stopwords
: Provide a list of words to exclude from the word cloud.
Other Articles: